Use min with an iterator (for python 3 use items instead of iteritems); instead of lambda use the itemgetter from operator, which is faster than lambda. From operator import itemgetter minkey= min (d.iteritems , key=itemgetter (1)). The Python min function is used to find the lowest value in a list. The list of values can contain either strings or numbers. You may encounter a situation where you want to find the minimum or maximum value in a list or a string. For instance, you may be writing a program that finds the most expensive car sold at your dealership. Python min function examples. Let’s look at some examples of python min function. Min with string. When min function is used with string argument, the character with minimum unicode value is returned. S = 'abcC' print(min(s)) for c in s: print(c, 'unicode value =', ord(c)) Output. Get the minimum value of all the column in python pandas: # get the minimum values of all the column in dataframe df.min This gives the list of all the column names and its minimum value, so the output will be. Get the minimum value of a specific column in python pandas: Example 1: # get the minimum value of the column 'Age' df'Age'.min. Python min and max are built-in functions in python which returns the smallest number and the largest number of the list respectively, as the output. Python min can also be used to find the smaller one in the comparison of two variables or lists.
Groupby minimum in pandas python can be accomplished by groupby() function. Groupby minimum of multiple column and single column in pandas is accomplished by multiple ways some among them are groupby() function and aggregate() function. let’s see how to
- Groupby single column in pandas – groupby minimum
- Groupby multiple columns in pandas – groupby minimum
- Groupby minimum using aggregate() function
- Groupby minimum using pivot() function.
- using reset_index() function for groupby multiple columns and single columns

First let’s create a dataframe
df1 will be
Groupby single column – groupby min pandas python:
groupby() function takes up the column name as argument followed by min() function as shown below
We will groupby min with single column (State), so the result will be

using reset_index()

reset_index() function resets and provides the new index to the grouped by dataframe and makes them a proper dataframe structure
We will groupby min with “State” column along with the reset_index() will give a proper table structure , so the result will be
Groupby multiple columns – groupby min pandas python:
We will groupby min with State and Product columns, so the result will be

Groupby Min of multiple columns in pandas using reset_index()
reset_index() function resets and provides the new index to the grouped by dataframe and makes them a proper dataframe structure
Python Minecraft
We will groupby min with “Product” and “State” columns along with the reset_index() will give a proper table structure , so the result will be
Using aggregate() function:
agg() function takes ‘min’ as input which performs groupby min, reset_index() assigns the new index to the grouped by dataframe and makes them a proper dataframe structure
Python Min Max
We will compute groupby min using agg() function with “Product” and “State” columns along with the reset_index() will give a proper table structure , so the result will be

using Pivot() function :
You can use the pivot() functionality to arrange the data in a nice table.
groupby() function along with the pivot function() gives a nice table format as shown below
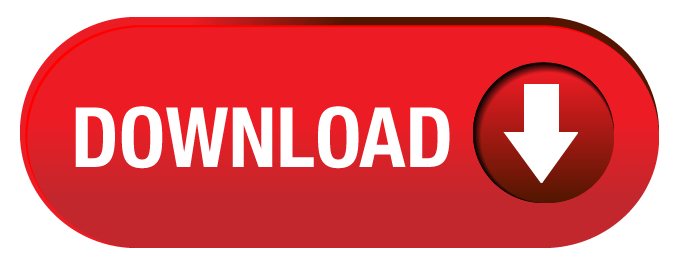